007 Interfaces
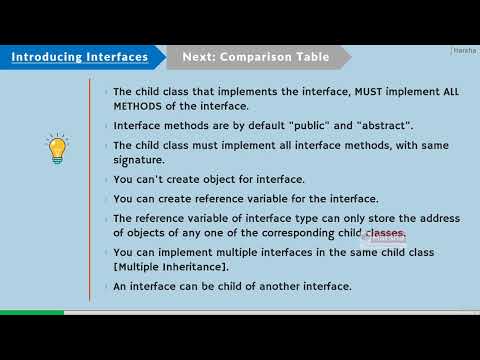
in this section we will explore through interfaces so first what is interface think of an interface is a pure abstract class which contains only the abstract methods in the abstract class you can Define normal methods and Abstract methods also but in the interface you can only Define the abstract methods you cannot Define any non-abstract methods in the interface so interface is a set of abstract methods that must be implemented by the child classes for example you take the classes called car and bus this to the child classes of vehicle because car is a vehicle and bus is also vehicle instead of creating the vehicle as an abst class you can also make the vehicle as interface generally interface names starts with the capital I here I for interface so the I vehicle is interface which is a parent of both classes that is car and bus which means that the interface contains the list of abstract methods which are common for both car and bus but the interface doesn't provide any implementation that means interface doesn't provide any method definition it will declare only the method names corresponding written types and parameters based on the signature of interface methods both child classes that is car and bus must Implement all the methods of the corresponding interface so coming to the syntax of interface first you should write a keyword called interface which says that you're creating an interface and then you should write the interface name and inside the interface body you can define a set of abstract methods without method definition so only you will mention the written type method name and parameters of the method so now method body and no access modifier the default access modifier of all the interface methods is public so this method is public by default you cannot change the access modere of the interface methods and then you can create a child class that implements the interface so for that case you will write colon which means that the child class inherits from the particular interface so by inheriting from the interface the CH class promises that all the interface methods must be implemented in the same child class so if the interface has 10 methods the child class must Implement all the 10 methods with the same parameters and same written type and same method name and the access modare must be public but the method body can be different you can implement the same interface in multiple child classes also for example the interface called I vehicle is implemented in both child classes that is car and bus so for the same interface method in one child class you can Define one method body in other child class you can Define other method body body so the method body that means the actual code of the method is a specific to the child class but the method signature that is the method name parameters and written type must be fixed which is defined in the interface so in this way the intention of an interface is that providing a set of methods that must be implemented in all the child classes of the interface so what is the benefit of interface assume some other developer is going to call the car class and bus class if he creates an object of car class and call the interface method the implementation of the method of cor class will be executed but in case if he creates an object of bus class and invoke the interface method then the method definition bus class will be executed so without worrying about the child class implementation he can invoke the interface method it is the responsibility of the child class to implement that method the set of rules of interface are the child class that implements the interface must Implement all the interface methods it cannot skip one or more methods interface methods are by default public and Abstract because in the abstract class we have created abstract methods with abstract keyword explicitly but in interface implicitly all the interface methods are public and Abstract while implementation in the child class the interface methods must be having same parameters and same written type as defined in the interface you cannot create an object for the interface just like we cannot create object of abstract class you can create reference variable for the interface in the reference variable of interface you can store the address of object of any one of the corresponding child classes of the same interface for example in the reference variable of I vehicle interface you can store the reference of object of either car class or bus class because car and bus are the child classes of the I vehicle interface and in the same way in the reference variable of I animal interface you can store the reference of object of either tiger class or dog Class A Child class can Implement multiple interfaces Whenever there are multiple parent interfaces it is called as multiple inheritance an interface can be a child interface of another parent interface it is called as interface inheritance so just like the child class is a child of parent class there can be a child interface that is a child of a parent interface now let us observe the comparison table that is abstract class versus interface the abstract class can inherit from other classes but interface cannot be inherited from other classes that means a class cannot be a parent of interface an abstract class can be a child of an interface in the same way an inter can be a child of another interface both absr classes and interfaces can be inherited that means a class can be a child of an abstract class or interface both abstract classes and interfaces cannot be instantiated that means you cannot create an object for abstract class or interface so the only difference between abstract class and interface is that abstract class can be inherited from other classes but interface cannot be inherited from other classes that means there can be a parent class for apps. class but there cannot be a parent class for interface now let us understand what members can be defined in the App Store class and interface phas first of all in the normal class we can Define almost all types of members except abstract methods means you can Define all types of members such as non-static Fields non-static methods and other non-static members and in the same way the static Fields static methods and other static members only abstract methods cannot be created in the normal class here non-static means instance members the non-static fields or the instance fields and inside the abstract class you can Define almost all types of members including abstract methods so you can Define non-static methods static methods nonstatic Fields static fields and other type of members such as property and Constructors but in the interface all these types of members cannot be created the only members you can create inside the interface is the non-static events abstract methods and non-static auto implemented properties except these three you cannot Define any other type of members in the interface and moreover by default all the interface methods are abstract methods implicitly even without writing the keyword abstract and coming to the auto implemented properties the intention of properties in the interface is that they must be implemented in the child class so the properties in the interface are not implemented by default and we will understand about events in the latter sections let's Implement interface practically now I would like to convert the abstract class called employee as interface so that I can declare only the properties and Abstract methods in the interface the same properties and methods must be implemented in all the corresponding child classes that is manager and salesman so the intention of an interface is to only provide the list of properties and methods that must be implemented in the corresponding child classes it forces the child class to have those properties and methods the benefit is that while invoking the child class the developer need not worry whether those properties and methods are created in the child class or not because the child classes are forced to have those properties and methods by interfaces so let us convert the abstr class as interface interface cannot contain Fields interface cannot contain Constructors interface can contain only abstract methods that is for example get insurance amount and all the interface methods are by default public and we cannot Define the same explicitly and all interface methods are by default abstract so only written type method name and parameters is a sufficient Syntax for creating the interface and now coming to the auto implemented properties in the Auto implemented properties of interface we cannot write actual definition of the set accessor and get accessor alternatively we should write the semicolon here because the definition of set and get accessors are written in the child class while implementation of the interface and interface members are by default public we cannot explicitly Define the same and same syntax is followed in all the remaining properties also so this is the overall definition of interface and it is recommended to prefix I before the interface name it is not compulsory but strongly recommended and it is better to rename the file also instead of employee it is better to make it as I employee so right click rename and specify IM employee. CS so now the I employee interface is ready so this interface defines the properties and methods that must exist in all types of employees that is manager or salesman but the interface doesn't worry about the implementation of these properties and methods it is led to the child class itself whereas the child classes such as manager class must promise that it will Implement all the properties and methods of the interface so manager is one of the child classes that implements the I employee interface so I am writing I employee I employee is the parent interface manager is the child class it must Implement all the properties and methods of the interface that is EMP ID EMP name and location so first I am creating the private fields for the same that is underscore EMP ID underscore EMP name and underscore location and you must implement the properties in the same way how we have created in the abst class we should Define the actual body of the set and get accessors so in this case the intention of interface is that rather than providing ready made properties and methods it is forcing the child classes to define a specific set of properties and methods and while W ring the interface methods you need not use the W keyword whereas you must use the wed keyword while implementation of abstract methods of abstract class but it is not required for interface so rather than this is calling method writing you can call it as implementation because the word overriding refers to extending the existing method but there is no method definition in the interface method right so you're not extending this you're following this so by following the existing method name creating a method is called as implementation and similarly the same type of properties and methods must be implemented in the child class also so I am copying this code into the salesman class so here also we have the fields that is underscore EMP idore EMP name and underscore location and the properties implementation what can be different between these two child classes is that different child classes can implement the properties or methods in different ways for example as per the rules of manager class the employee ID must be in between 1,1 and 2,000 so in the set accessor of empid property in the manager class I'm going to add the condition saying that if the value is greater than or equal to 10,000 and less than or equal to 2,000 then only we have to assign the value into the underscore empid field but this validation may be differently implemented in the other child class that is salesman suppose here I am writing the condition as it should be between 500 and 1,000 so this is the real benefit of interface interface stick to the point that every child class must contain the EMP ID property but the interface doesn't involve in the implementation of the property or method so it is the freedom of the child classes to implement the property and Method on its own so you can Define one logic in the child class one and you can Define other logic in the other child class and in the same way in the methods also you can maintain some difference for example in the interface method of the child class one I am wring the output as th000 and even this text message also can be changed and in the interface method of the other child class that is salesman I am wring the value as 500 so it is up to the CH class how it will implement the same method means the method body can differ among the interface methods of both child classes and this is called method implementation and you need not use the wed keyword and now coming to the program class that is the console application here instead of creating a reference variable for the child class itself so in the logic of main method almost there is no much difference so you are creating an object of the manager class and working with the same and in the same way you're working with the salesman class object and specify the interface name in the inheritance of the salesman class and since the parent is in interface you need not explicitly call the parent class Constructor because there is no parent class Constructor here right the parentage interface and in the same way in the Constructor of salesman class also you need not call the parent class interface but in this case you must initialize all the values in the child class Constructor itself so in the salesman class of the class Library we have written the code for initializing the EMP ID EMP name and location fields and in the same way in the manager class also initialize the values of EMP ID EMP name and location in the Constructor and in the parent interface since there is no any field called underscore location you need do and cannot use the base keyword to call the field because underscore field is a part of the same class itself it is not a part of the parent interface it is working normally as expected like before there is no particular change in the output so overall in real time projects when to use interfaces so whenever you find out a parent such as animal is a parent of tiger dog Etc it is better to make the parent either as abstract class or interface so when exactly you will go for interface and when exactly you will go for abstract class whenever you want to Define some methods as abstract methods and remaining methods as non-abstract methods that means for some methods you want to define the method body but some other methods you don't want to define the method body so you have a combination of abstract methods and non-abstract methods in that case better to go for abstract class in that case the parent class that is abstract class provid provides the implemented methods and non-implemented methods also to the child classes in that case the child classes of abstract class can call all the non-abstract methods of the abstract class and at the same time the child classes must Implement all the abstract methods of the abstract class but when will you use the interfaces whenever in the p you want to provide only the abstract methods but don't want to provide any non-abstract methods and other fields or Constructors in that case go for interface in this case interface provides only abstract methods to the child classes all the child classes of the interface must Implement all the abstract methods of the interface and the developer who is going to call the child class need not worry whether the properties and methods are implemented in the child class it is the guarantee provided by interface because the child class of interface must Implement all the properties and methods of the interface so without a doubt the developer can call any interface property and interface method from the child class object this is the real benefit of interface this is understanding the interfaces in C
2024-12-21 21:19