Aston Technologies | Minneapolis Tech Talks
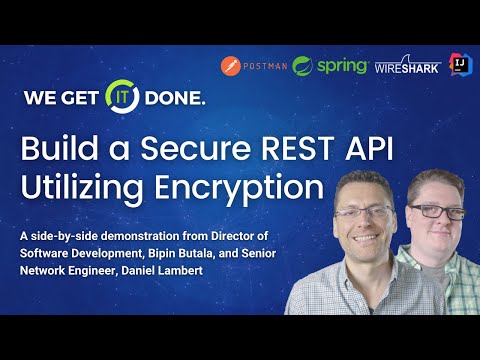
relational database so i'm going to do that i'm also going to use a tool called lombok just to prevent me from having to create getters and setters um now as you can see it's it's starting to bark at me a little bit here it says it persists a persistent entity should have a primary key so then we can put any number of uh fields and data types we want inside this entity but we need at least one that's going to refer to the primary key of that table if we look back here in account maybe you can't see it but i've created the and we can see the ddl here um so this is just the the sequel to create the table um and i've create i've marked the account number as the primary key for this table so that's what's going to be used to look things up um it's also going to be indexed by default so let's close some of these things um okay so i need to and that was a string um and i called it user no i called it a count number and that is going to be annotated with at id so what i'm doing here is i'm telling uh spring data and jpa that this field right here is the one that i want you to treat as the identifier i just need to bring in the import um okay and really all we need to do after that is just match the uh fields we have account owner balance password username account owner password username are strings balance is going to be a double or a decimal um so just go ahead and do that so i have string account owner i've got string i think i call it username string uh password um and then a double of the balance so um this this will represent all of the fields and this is sort of a java container that we can put the data that we get from our database into and it'll serialize it into json going up eventually and also we can use it to you know write to the database as well which we're not going to cover in this one but um just be aware that's there so i have this container now really all i have to do outside of that is create an interface so this is the real power of spring data spring data will give you all basic relational database crud operations by default by simply extending uh an already existing interface i'm going to call this thing account repository and all i need to do here is extend crud repository which is a spring data interface that allows that has pre-built you're i'm just going to jump into there pre-built methods for all the common kind of database interactions that you're going to do so this is like all the the base ones and if you need to create more custom ones you can easily do that within the interface um but and and to do this we need to tell the crowd repository just two things we need to tell it that uh the type so that's gonna be the account entity that we just created and we need to tell it the data type of the id so that was not an integer that was a or was it an integer it should be an integer but i think i called it a string here there we go all right um so with those two things in place i now have all basic create retrieve update and delete functionality available in my database or in my uh sorry in my spring application so i'm gonna go ahead and fire this up um there's a couple ways you can do it uh using intellij this is the main entry point so i could have just launched it from here otherwise it's probably too small for you to see but there's a little play button up top so it's saying hey we can't i don't know the url data source isn't there that's because we need to tell not only tell uh the application what the table looks like we also need to give it the connection string to get us into that so i'm just going to punch in spring data source and we're using the mysql driver and the url is going to be jdbc sql local host 3306 and bank is the name of the thing that we create the schema data source username i think i should put root spring data source password so with that in our application properties which is which is identical to what i used to connect to the database here in the ide the application should have enough information to go ahead and start so i'm going to fire it off again hopefully things bounce up so okay so the application started it's not doing anything yet let's uh let's create a little test just to make sure that we can see that data that is available so if we want to use that repository that i just created we use a concept called dependency injection to auto wire that in um account repository now this is another this is really the core of what spring is it's a dependency injection engine and the spring context its primary function is to figure out which dependencies to use um in in which context so with that um i am going to create a test and void db connection we'll just call it i just want to have a little sanity check that things are actually i can actually get to the data that's in the sql database from the code here um can we see that okay too one more um so account repository has a bunch of helpful methods as i showed you before um and one of those is find all and this will just retrieve everything in the database it's a little bit um the nuclear option for what we're doing here if you say had 10 billion records in your database it would not be a great thing to run but since we only have four i am going to just go ahead and run stick with that so if we run this test hopefully what i should see print it out to the console below here is all of the data serialized there it was for a second there popped up as you can see i'm getting that data now into my test which means that the connection between my database here and my repository layer in my application is good to go so with that in place i'm now going to set up the ap or the rest api endpoints so i'm going to make url paths that will then return return turn some of that data so um to do that i'm going to create another file here java class i'm going to call this account rest what you see it is account rest controller and to tag this as a rest controller you intuitively name it or annotate the class as rest controller which means that spring is going to inject this controller as a as a mapping between urls and java code so we are going to need our repository in there so i'm just going to wire it in just like we did in the test itself um and then i am going to create an endpoint so an endpoint or url or a resource is going to get um is going to be the suffix to the server so at www.google.com is going to be my server slash gmail that would be my endpoint right so i'm just going to create a little dummy one here it's just going to be called let's call it hello and then let's see public it's going to return just a string and we'll say greeting what you name the method doesn't really matter i'm not going to pass anything in right now and i'm simply going to return hello aston tech talk audience okay with that in place i am going to restart oops that's just going to run the test okay so with this up and running and what you uh what you don't see here but i'm going to move to a little more detailed view of this again just to review we we built we use jdpc and spring data going across port 3306 to connect to our uh sql database server there um and then inside of spring by default there is a web server if we bring in the web package which i did and that's tomcat tomcat is a very popular server it's actually built into a lot of cisco products that are out there as well as tons of other things and it's a really streamlined web server that will run java so you don't really need to interact with it specifically but uh you know it can run as a standalone application but in the case of spring boot it lives inside of uh the application itself which makes it very convenient and easy to spin up i don't have to install some server and then put my code on that server it's all baked right into the code um so uh yeah so that's what i wanted to talk about tomcat is our web server um and it's not really it's kind of again under the hood or behind the scenes doing things so our app is up and running uh the url is hello i for the sake of this demonstration of running everything on localhost but of course in a real world that we push to an actual server maybe in aws or somewhere out there in the wild so i am still on http because that's what comes by default um localhost i called it hello i believe hello okay so we've just created our first endpoint we've got our web server up um you know it's not doing much but you know just to kind of review we are going to now take care of this kind of connection between uh the data layer and the api layer so i'm going to want to create an endpoint that will take an account number and then just give me that information back so i need a way to get data into my endpoint so let's just modify our example a little bit here and pass in what's called a path variable or a path parameter which means what i call this account number that's right so this is going to allow me to take data in the url and pass it into this method here so i'm going to annotate path variable and it's going to be a that's going to be an integer and it's going to be called we'll call it a count number just for consistency um no i don't want to do that actually right now i'm just doing string name name and then instead of this we're just going to use we're just going to concatenate some strings together to uh to prove that we're taking information from our url it's getting into our method put smash together between hello and exclamation more marks and then return back so um restart real quick make sure that's up and running um while that's going so hello now is expecting a parameter at the end and if i say dan hit enter it'll take that information displayed so again not very wowing or whatever but just kind of showing you how we get data from the url or uh the request body or maybe query parameters into our controller method so all right let's do something that's got some actual meat to it so i'm going to use git mapping make another endpoint um and this endpoint is going to be slash account slash and then i'm going to put in curly brackets here count number um it's going to be very similar to the last method we created oops that's what i want to do we're going to name this thing public or it's a public method and we're going to use a response entity which is a kind of a wrapper for the response it allows us to add custom headers change the response code from 200 to 201 to whatever 400 500 for errors and stuff like that um so we're going to use a response entity and inside of it is going to be in the body of that response is going to be an account hopefully if we find it from the account number um so we'll just say get count details and this is going to have a path variable uh and that's going to be an integer and it will be called account number again it doesn't really matter what i name it here but for consistency i'm going to stick with that so now we're going to return not a string but we're going to return a response entity with a builder okay okay is a 200 response and in the body of this response i am going to do use the account repository find by id and then pass the id that's coming from the path parameter into my repository method this returns an optional and just for the sake of this demonstration i'm just going to retrieve it an optional is a another wrapper in case it doesn't find it you won't get a null pointer but that's for another discussion so with this in place i've created an endpoint account slash whatever number we put in place and it's going to pass that number down to the repository that we created which is then going to go into the database query for that get the data back serialize it into json and send it back up right so again just to look i'll restart it while this is again um you know the point here is to be able to get to that bank account data from a browser um across we're still on 80 here on across normal http unencrypted uh traffic um and should see the data so um in that i i put the endpoint as account um and then 1541 was one of the accounts oops it likes to default to https so sweet so there is our data um so we have created an api that goes all the way through that you know that you can an api running on tomcat that's connected to a relational database mysql and if we pass an account number it'll retrieve that record in the database in my xcode project here too for that iphone app i'm just hitting localhost account and then passing in a number that i'm getting from this input field here so now 1541 if i run account balance it actually connects out and gets that data um so with that uh kind of all built and in place um i'm gonna hand it over to dan who's gonna show you why what i did right here is totally susceptible to some sort of some sort of packet capture or nefarious man in the middle hello everybody my name is daniel lambert or dan lambert is generally what i go by i'm a network engineer for aston technologies and my goal here is to explain on the most basic exactly how a network actually works and how we can use a tool called wireshark which is able to actually look inside of a data stream between you know two computers that are communicating and show us the literal ones and zeros that are transmitted to and from the uh the client and the server in this case so we already have wireshark pulled up right here and actually i wanted to start that off by showing that this is a free and open source tool that we can just go to www.wire it's not com though i'm a liar wireshark.org and so from this site right here you can just simply go to the download option and you know whatever operating system you're using it'll go ahead and give you a nice little indicator of what you should download in on your machine in order to run wireshark now wireshark itself is simply just a tool to actually view packet captures and what a packet capture is is a literal just it's an organization of the actual ones and zeros that are sent from one network interface to talk to another one when you actually install this it's going to want to install a bunch of other stuff on your computer and a windows computer it's called win pcap i actually don't know what it's called inside of an apple computer but those extra drivers are the actual software that listens on the network interface cards inside of your computer and actually tracks those ones and zeros and is capable of saving them as a p-cap file they're usually just dot p-cap if you ever are viewing them in the real world and then wireshark is the tool that can open that dot p-cap file in order for you to actually see the ones and zeros so wireshark is kind of like notepad pcap files are kind of like like dot text files so when we get wireshark open like this we have a list of every single interface that's on our computer we don't just take a packet capture from a computer but we rather take packet captures captures from network interfaces themselves and in this case we'll probably be focusing mostly on the loopback in order to see the the traffic the bipin created today because he's hosting the web server inside of this computer and you know we're using this computer to access that web server so none of this data actually ends up out on the actual network but before i do that i'll go ahead and take a packet capture of just some general wi-fi traffic and show you guys what it will look like you know in the wild on the computer so i'm going to remove any filters that are currently on there i select the wi-fi interface and just by hitting the little blue shark fan hump at the top i actually begin the packet capture where we can start to see packets come through it's broken up into three separate windows the top window here is an ordered list of all of our different packets that are coming in from the network they're ordered from the first packet since i started the packet capture that was red and it just incrementally counts up one by one it tells us the time um since we last heard our last packet or since the beginning of the packet capture and gives us kind of a plethora of other information we're not going to try to teach everything about wireshark today because since we're actually literally looking at the ones and zeroes you'd have to literally understand every single protocol that's running on a network in order to really get it and honestly i don't know all that stuff so we're just going to focus on the things here that we do want to touch on the middle window is actually going to break out and understand or interpret the data that was pertaining to each individual packet so i can select different packets and you'll see the bottom two windows are changing between different data types if we focus on the bottom here we can see the actual literal ones and zeros as they transmit across our network uh something that i think is kind of an important takeaway or at least was a big aha moment for me when i was learning networking is that all data on networks are transmitted linearly the ones and zeros have to you know there's a first one and zero that gets sent and then each one and zero afterwards linearly came after that there wasn't like two percent in parallel and then every so chunk of these data say 1500 bytes worth of ones and zeros becomes what we would call a packet and those packets are sent linearly just as we're looking at the top so the ones and zeros are you know everything can just be strung along into like one big long chain so those ones and zeros though are pretty hard for a human to interpret technically if you were good enough you could look at these ones and zeros and start to be like okay i see everything that's inside of this packet because i just know how these protocols work so well but i would say the vast majority of human beings out there can't do that and so wireshark is a tool that allows us to actually get some human readable data from this and that's what this middle window is all about there ends up being a few different drop-downs that we have here and each of these drop-downs is a different portion of the packet in fact if i click this part right here where it says ethernet and you'll see that got highlighted in red down in the bottom portion it's highlighted the ones and zeros that make up that part of the packet that was sent if i then click a different section inside of here you'll see it'll highlight those and you may even pick up as i click down these one by one it's jumping around so it makes it a little hard to see but you know it starts with the ethernet header those are the very first ones and zeros then it goes to the next header the next layer we sometimes call it inside the packet which happens and starts immediately after the previous layer and so on and so forth until we get to the entire contents of the packet that's sent so obviously by default you know packets don't have human readable data and that really is wireshark's trick in order to have that middle pane show us everything it uses a tool called dissectors in order to do that we can actually take a lip look at all of them by going to wireshark preferences and we can take a look at protocols right here you know there's a giant list of protocols that exist inside of here and they all have various you know parameters you might be able to modify about them but any you know protocol that's been uh had a dissector we would call it created for wireshark when you then run wireshark to read these ones and zeros it will match any ones and zeros it sees to the relevant dissector and then display that data in a way that we can actually interpret it so maybe just to walk through one of these packets we have the very first packet here and we open up the very first part of this just the the frame section and we'll see it highlights all of the bits there the first section here isn't actually any of the ones and zeros but it rather talks mostly about time it'll tell you the first layer of encapsulation that's used on the packet so it knows how to start reading the very first ones and zeros and then it tells us stuff like the timing of when the packet was actually sent epoch time really commonly used in networking or really any kind of computing it's counting up from i believe january 1st 1970 um and so if you're looking at maybe multiple different packet captures and you're trying to compare times epoch time can be a reliable point to compare against we would call this in networking the layer one portion of the packet layer one refers to the physical layer and if someone you ever hear network engineer say oh we're having a layer one problem it means something is physically wrong with stuff it's not that anything was configured it's like someone literally went and unplugged the cable or clipped to the cable or intentionally damaged the device by pouring water on it so that's the first layer of what we would call the osi model now as you get deeper into a network each of these different dissectors or layers inside of here we go deeper into that osi model which has a total of seven layers although we kind of ignore two of them but the very next layer is layer two and in this case it's called ethernet and it's the very first set of ones and zeros now by default wireshark knows to interpret these as ones and zeros or as ethernet because these were captured off of an ethernet interface so a you know you have different types of layer 2 protocols out there although the vast majority of what a network engineer a data network engineer is going to focus on are all ethernet networks the actual protocol that makes up the first ones and zeros is pretty much universally dictated by the interface that you're plugging into on the device is this an ethernet interface or is it a fiber channel interface and if we expand the contents of what's inside of the ethernet interface we'll see this isn't actually displaying all that much data ethernet it's a pretty simple protocol and the relevant information is actually quite short we have a source and destination mac address which again by clicking them inside of here we can highlight the actual ones and zeros down there that make up that actual data um and these are hardware addresses these are addresses that are physically on the network interface card of either the sending or receiving device that we're sending to um they can be a little bit thought of as like your name right so as a human being we have a name mac addresses are sort of like the network interface card's name so that we can identify it when we're looking inside of here and it's also used for you know actually being able to know where to send packets but you'll notice this next field right below it where it says type and that's the field i really wanted to focus on after you know wireshark's dissector has gone through all of the ones and zeros that make up ethernet it's exactly 64 bytes for ethernet it needs to know how to think about the next layer of the packet that it's reading and the way that it knows that is there'll be a type field in the ethernet header that has two bytes given as we can see from the highlighted red parts of how to interpret the next zeros and ones that come inside of this packet in this case we can see it's using ipv4 or we could call it ip for the most part there are a couple other versions but if we open the ip header we've now moved to the next layer of a network engineer's osi model we've gone from layer two into layer three layer three is always going to be encapsulated inside of some layer two protocol in this case ethernet so the ip header here has a little bit more information there's a little bit more that goes into ip but you know the main thing i want to focus on here is that we have a source and destination ip address the source address is quite literally the address that's on the computer that we're sourcing the traffic from and then the destination address is quite literally the thing that we're trying to talk to is ip address now the difference between a mac address and an ip address is pretty simple that's more like your home address it can change and as the physical piece of hardware moves to different networks and moves around the world you're not always going to have the same ip address but typically you'll keep the same mac address and then just like the previous protocol of ethernet we have a field in here this time called protocol not to be confusing instead of type but that tells you know not just wireshark but the receiving computer how to interpret the ones and zeroes that happen in the next section of the packet so it counts through all of the layer three packets and it's like okay the next layer is going to be interpreted as a tcp packet open up the contents of this we can see all of the different things that make up the tcp packet the key thing in tcp packet that we can probably correlate to bippin's parts is the source and destination ports that are chosen the currently his web server that he built is listening on tcp port 80. and so the source port or the destination port when we look at his traffic we'll actually see is going to be using port 80 and then there'll be always a source port from the client that's sending it ports aren't one-sided now in the ccp field you don't actually have a type or a protocol field so you know our dissectors inside a wireshark or a receiving computer doesn't necessarily know how to read that next layer although clearly it does here because it's automatically figured out that it's using the tls protocol so the way that that happens for wireshark is it's actually implied it saw that the conversation was using a particular port number in this case 443 and by and large that's the standard that we would use for tls traffic on any network and the by just nature of that being four for three wireshark is going to jump to conclusions and try to interpret that as a tls packet on rare occasions it would happen where the ports and the dissector don't align and you get a bunch of gibberish as a result but you can go into that protocol fields i was showing you before in preferences and you can actually modify things to get to be like okay this protocol is actually running on this port or vice versa so that's how wireshark figures it out your computer figures it out literally by the configurations that would exist on something like tomcat when he actually stood up the web server when he configures that and says listen on port 80. he's told that server that computer and that operating system to actually open up port 80 on that computer and if you ever hear any traffic come into that interpret it this very specific way since it's an http web server that is how that server would then know to interpret the next bits zeros and ones in that packet as the next layer inside of the wireshark capture or inside of the packet so that's kind of an overview of generally we've talked about kind of the osi model and the different layers i guess i skipped tcp it's the layer four from ips layer three and then technically tls skips five and six and goes directly to seven that happens a lot where we don't really use the osi layers five or six at all um so let's stop this packet capture and start another one where we're actually going to try to see the data that bipin created in his uh and his little program so this time i'm going to choose to stiff the packets off of the loopback interface rather than the actual wi-fi interface that was inside of this computer and the purpose of that is that again the web server is inside of this computer we're going to be testing using this computer all of the packets are contained inside of this computer and so if we just sniff the wi-fi interface none of those packets actually are going to get transmitted out of the computer so it's not really you know useful here but if we go ahead and start the packet capture in the loopback interface we can go ahead and go and create some data so here i could just refresh this page and go to it there like that and in theory i have some new packets which i do um we'll show again that we can also do it with the phone application here in just a second to kind of show they work the same way now in the top field of wireshark here we have the ability to apply our own filters i mean of course i could just start scrolling through this and look for whatever data i actually want and in this case there's not much there so it'd be pretty easy but normally network engineers are getting packet captures that are thousands tens or hundreds of thousands of packets long and you're not just going to scroll around until you see the one you want so this filter page at the top allows us to look for specific things inside of here and in this case i know using he is using an http application so i'm going to type http in the top and hit enter it's actually pretty nice how easy a lot of these filters are inside a wireshark it's you think what it is you type it in and it happens to be a real filter i feel it's pretty well thought out and then we can see two packets here that these do actually represent i believe the traffic that we just generated so i can go ahead and select this whole thing and i can hit follow this tcp stream it'll pull up some data here we'll actually be able to see immediately that we're already seeing that data in plain text as it would have happened across the network this little window is just a summary that wireshark creates for us the red is the packets that our client sent the blue is the packets that our client received and it'll just show us the raw data as it sought but we can also just look at it inside of here and now we're following the specific tcp stream now getting into a little bit deeper into the network side these first three packets that we'll see that are noted as a syn packet a syn ack packet in an ack packet is a unique property of the tcp protocol without going into depth on it tcp is a protocol used by networks such that if ever a packet is lost across the network and you know random things can happen a computer didn't function quite properly or something is causing packets to not make it all the way to the endpoint successfully tcp can detect that those packets were missed and then it's capable of resending all the packets that were missed um in order to do that though it has to create a session or a socket we sometimes call it and in order to do that this three-way handshake must be completed so anytime you're looking at any web traffic or any tcp traffic at all you're always going to see it start off with a sin a synack and an ack packet in fact if you ever look at tcp traffic inside of a wireshark capture and it doesn't have that then you didn't catch the very start of the conversation you're seeing the tcp you started the packet capture after the tcp conversation had already gone had gone online now you can see in the protocol field it identifies each of these packets as tcp packets and if we kind of shrink this middle section again and get rid of this bottom section it's not as important now we can see that we only go up to the tcp field here there is no extra layer on top of this packet the packet actually ends at this point because it's just part of tcp's three-way handshake but after that completes we do get to an http packet as i have highlighted here and we get that additional layer that's added now here you're going to see that there was a get request sent this is part of uh you know the api that bippin created um it's used heavily by the http protocol to request files from different systems and we can see the request uri of account slash 150 or 1541 which i believe if you have that pulled up somewhere your ide to the right all the way huh we won't worry about that for right now i'll let pippin re show that when he's on there but this section right here where we see the account slash 151 41 that is exactly what we put in the top field after local host up there and that was directly written into his programming language that that's what the or in this ide what the account was actually or the program is actually listening for so inside of wireshark we send that request we see that uri and then wireshark sends a few more packets and we finally get down to this 200 packet this is the final receipt packet that is returning all of the data that we requested now if you look at this section right here that i currently have highlighted you can see it's two reassembled tcp segments so ultimately all this is trying to show is that often times you're trying to send things that won't fit inside of a single packet on the network maybe this content isn't too large but if you're sending a 4k image or a video file of some sort there's no way you're getting that entire thing inside of a single packet and slamming it through the network and so it'll get broken up into lots of little packets and the wireshark figures that out for you it's part of the dissector and it will go ahead and figure out hey look i have those two tcp packets above specifically number 85 and 87 which you can see here is 85 and then 87 so when we're actually on it's like i've reassembled that data to represent you know the actual data that was flowing um between the server and client at that moment and we can scroll down through all of this close the http layer and then we can see the actual content of the payload of the packet at this point to a network engineer we don't really care what's inside of the json stuff because we're usually operating on the first four layers of a network but you know we can see the unencrypted javascript or json formatted data in here and you can see all of the different you know key value pairs that were inside of that and we could see we can get the bank account balance we could get the password and let's say i had a really large packet capture and i was trying to target a specific person i could go through the effort of taking this figure right here going i want to apply this filter as selected and up here at the top it's automatically just generated what the filter would look like for me to search for a json formatted string in that packet and then i could just go ahead and change what's inside of the the quotes right here to be any name i wanted and then i could target a specific person if i was trying to look for their data moral of the story though wireshark well super useful to a network engineer when they're troubleshooting things on the network is also super useful to a malicious attacker where if they're able to be anywhere inside of your network where they could plug in a computer and start to sniff the packets like we're seeing here if you don't encrypt the data they would be able to see it in plain text just like this and i will hand it over to bippin now to talk a little bit about securing that all right there so i was just saying that a common scenario is one that we've just encountered here as far as you know i'm a developer building an api for our bank's new iphone app or mobile app um and i build it and then the the networking team or security team says this is completely not okay because you have it completely unencrypted and anybody can see this information and we'll get sued and go out of business and just got our mobile app off the ground so um so in order to do that i don't need to change anything about my api but more actually go back to our diagram more so i need to change the protocol that tomcat is listening on right by default tomcat was listening to http traffic um i and by default it's on 8080. i overrode that to port 80. but i need to
continue to configure tomcat to operate uh using ssl or tls um on https on port 443. so first off i am going to go into my application properties here and i'm going to change from port 80 to port 443 it would be great if that was all you needed to do but um unfortunately that uh isn't all because i not only do i need to change ports i also need to enable uh ssl so okay another super easy thing to do but beyond that i need to create some sort of secret some sort of very complex key uh if you will that can be shared and will allow the information to be encrypted and then on the other side decrypted so uh the jdk or the java development kit which is kind of the underlying code language that everything that i'm doing here in spring and intellij runs off of has a tool uh that's built in called key tool if you install the jdk you get key tool with it and just by running it a command line tool i can pass it a bunch of options one of the things that ke2 will do is it'll generate a key pair and a certificate for me and this is i'm what i'm creating now is called a self-signed certificate right um it's not correlated to any certificate authority so your browsers would still be confused by it but the traffic on it will be encrypted so it's a bit of a long command uh but you know uh this information is readily available on the internet and also uh there'll be uh links to everything that i've done and some of the things that dan has done too in uh the the meeting notes afterwards so um i'm going to give this key pair an alias i'm just going to call it aston i'm going to give it an algorithm rsa as far as i know this is one of the most common algorithms for encryption um i'm going to give it a key size just go with 2048 i believe the larger the key size the harder it is to uh crack um i'm going to pick a type there's going to be a pks file a pka pkcs12 um and then we should give this file a name i'm gonna call it astonp12 um and then just a span a validity of 365 times 10 so 10 years um so i'm going to hit that it's going to prompt me for some information i'm just going to create a dummy password password um and now we want some meta data to uh bundle into the key pair so i'm just going to give it this is where i'm signing it my dev organization aston uh minneapolis minnesota okay and then this is looks good it's going to crunch away and now i should have a p12 file it says it's a binary file do you want to see it sure yes so it's just a bunch of garbage right not very useful to us um but inside of that we just need to now attach this p12 file to uh our tomcat server so it knows whenever it's listening on port 443 any requests that come in to encrypt them on their way out so i've created this self-signed key pair and i just need to move it it showed up down here i'm just going to create a folder inside of resources called keystore and i'm going to move my p12 file in there now i don't know if you could see but i was i was prompted to create a password and an alias for that key store this p12 file if you will um and i just need to tell uh tomcat first a few things i need to tell it the key store which lives in our class path um i put it in keystore aston p12 i need to tell it the uh store type which was pkc i need to tell it the alias which i just called aston and the password the key store password oops which i just called password so it's similar to our database connection i'm just passing in some information that relates to that new key that i gave or that i created this i'm sorry this uh key pair p12 file that i created so with these pieces in place um i switched ports to 443 and enabled it um i'm gonna turn it on uh and then gave it this information about the p12 file that i just created and so now hopefully when i restart we're moving from port 80 to port 443 which is the default for https traffic um and it didn't have any problems starting up as you can see it's running uh https on port 443 so let's hop over to here now instead of http i'm going to do https localhost accounts account so now why are now firefox is barking we say hey this is not secure this this potential security risk right um i'll go look at it local hosts use an unbalanced security certificate if i wanted to get around this i'd have to register my certificate with a certificate authority um i can't remember what some of the names of those are but it's basically a service that you that is you know held in high authority that you pay to register your certificate with and you'll see in places like wireshark i hope wireshark has it but um that the connection is secure uh and that the uh certificate matches the name but since i'm running on localhost it's a self-signed certificate um i'm not going to get that here but i'm going to go ahead and accept the risk and continue because i know what it is but what you will see is if i go here connection is not secure but if i look at more information i'll see that the technical details probably very hard to see i don't know how to change the font here but um that the connection is encrypted and it talks about the tls protocol for that so um you know here i am uh doing my new development and the network team got on my case about my unencrypted api um so here it is i've created my encrypted api so hopefully this should stand the test of um dan's packet capture here and again i'm running on 443 so we'll see what happens from there all right so let's see if we can see that traffic now just add a little bit to what bipping was just describing about the certificates which is all super true about getting the certificate actually registered with the certificate authority the ultimate thing that's happening there is well the certificate is a multi-purpose tool it's actually used as a public key and part of the encryption of the packets that we're encrypting here it's also used as an identifier so it's a little bit like a driver's license to use another human metaphor and if you don't get your driver's license registered like from the dmv then people probably aren't going to trust it basically what pippin did when he self signed a certificate on the computer as he went to his home printer and printed out an id and if he tries to go by alcohol with that it's probably going to get turned down because they don't trust that authority to actually authenticate that so getting it registered with a with one of the profound authorities like verisign or even google does some or it looked like cloud of cloudflare was the one that did wireshark these have gone through significant auditing process with all the different operating systems and browsers to be trusted sources by default and so if you pay them get your certificate trusted they're obviously going to validate that you're actually allowed to have it the same way the dmv would validate you are who you say you are before giving you an id then any computer that goes to it could reach it without that certificate error so coming back here to wireshark and one thing i actually just want to confirm before we get too far in here is that i don't have a key currently mapped in here one second there are too many protocols in here all right perfect so i'm going to stop this packet capture we're still on the loopback so i'm just going to restart another packet capture directly in here i'm going to replace the http filter back on here and we're just going to go ahead and hit go on that continue without saving all right i'm just gonna go and try to generate some traffic right here that is still https as we saw before and if i come back to wireshark it doesn't see anything this time so what's happened is wireshark has that dissector that's looking for http traffic and technically it's looking for port 80 but even then it would not be able to see any portion of the http header at this point so let's clear the filter and actually try to look for it well what's some information we know about it we know that his destination port for the server he has built is using tcp port 443 so what i'm going to do is i'm going to expand the tcp part here select the destination port and i'm going to hit the apply the filter is selected there and now i have a new filter that i can change the number on be like i want this to be four four three and i hit enter and so now i'm looking at traffic that specifically is coming from our computer to the web server on port 443 we can see the source and destination those ip addresses are local host ips that's the ip your computer will forward traffic to when it's forwarding it to itself and that's why we see the source and the destination is the same here the source port it's kind of randomly selected by your operating system it's called the ephemeral port and then 443. so from looking at this i'm actually going to just right-click one of these and go to follow this tcp stream like we did before and you'll see we kind of get a bunch of gibberish this time so this time i'm not actually just plainly seeing the data immediately when i get this filter up and if i actually look inside of this well i still see the tcp three-way handshake you know this is kind of an important thing to note your ethernet layer your ip layer and your tcp layer those don't get encrypted if you were to encrypt that data you'd have no idea how to get it there right you might be sending a really important piece of data through the mail that you don't really want anyone get the hands on but if you just scribble all over where you're supposed to write the address it's not going to get there so same concept here we have to leave that stuff unencrypted but that final layer the layer 7 where http happens well we're not going to be able to see that we see a tls handshake happen here that is the security protocol that was used to encrypt the data here um part of https would use tls or ssl encryption and eventually we see it complete and then we just get these chunks that you know say application data and that's where the actual data is that we are sending but we can even use wireshark and drill all the way down into that tls layer like so oh boy clicking problems there we go then we have the encrypted data so that's about the bulk that we could see now now we trust in cryptography in general and that these different encryptions um are still working suitably and that they would haven't had some massive exploits such that a hacker could figure out a way to reverse engineer this but the modern protocols were on are and currently to public knowledge not easily exploitable and when it becomes public knowledge that one doesn't work we typically move to other versions that's actually how we ended up on tls 1.3 or 1.2 that we use now you know there were many predecessors before this but as exploits became public they would rework it and rebuild them such that there there weren't any and so if we were actually a man in the middle here plugging into some router on the internet or somewhere in the middle between where this traffic was traveling we would not be able to see the actual contents of the api that bippin was had created now there's one kind of catch here and wireshark is a useful tool obviously something can decrypt the data because the server receives the sends data the client receives the data and then it's able to actually see it in plain text and there are something called private keys there's a private key that's stored on your server that should never leave the server and no one should ever get it same thing on the client side when it goes to visit something it'll have its own private key that it'll generate and use in order to create this session now those are supposed to be exclusively local inside of your computer and you shouldn't be able to um you know some hacker listening in the middle of the network shouldn't be able to gain access to that but this is a good point to bring up the importance of not clicking phishing emails because if you click the link that you weren't supposed to click in an email like someone was trying to target you to gain access or be able to unencrypt your data simply clicking that leak could effectively forward your private keys to them and then if they got this data in the middle they'd be able to use that private key to unencrypt the data so how might that work if we stop this packet capture cancel we're going to find that terminal window again and that one right there perfect i'm going to run a script that pippin created beforehand we're not really going to go into the details of this that restarts the entire script for us and has also created this special file inside of the computer which we'll be able to point wireshark at which is our local private keys effectively to unencrypt the data if we go to preferences we go to protocols we scroll through two million of them we get to tls we can point to this pre-master secret file all right where is how am i going users aston developer there it is the ssl key file right here we're going to hit open and now we've told wireshark for its tls dissector to look for private keys inside of that file and so if we hit ok we're going to go ahead and start another packet capture right now continue without saving again this time i am just going to filter on http we scroll back over to this we try to regenerate that data https if everything worked we can now see the data playing text inside of there again so that data was still encrypted on the wire but if someone gained access to that secret key file or that yeah the secret key file and was able to plug it into wireshark this way just as before they'd be able to go to this packet scroll down and see all of the actual raw data that existed on that network again so be very careful clicking links that you don't don't really trust and i think that wraps up yeah thanks dan yeah no problem um um let's open up for any questions uh either here in the audience or out there and then i have some we have some uh challenge questions so um let's do the challenge questions first yeah okay cool so uh for a free yeti mug can someone tell me the command line tool that i use to generate the certificate and key pair okay all right fair enough can someone tell me as long as it's not up there um what the default port that uh tls or https runs on yeah no yeah close very close 443.
all right so then for my question wait for everyone down real quick all right so then my question is what is the name of the tool inside of wireshark that allows us to see the protocols in a human readable format what's the name of the file uh it's the what is the name of the tool or module or idea that wireshark uses that allows us to take the zeros and ones and print them out in a human readable format such that we can actually tell what's going on that's pretty useful known under that one the answer that one was dissector um what else do i got what is the name of the highest layer protocol that we usually are using on the network if when i was given the presentation it was the very first one that we talked about it's a layer two protocol what is the name that we of the protocol that we typically are using on a data network ethernet correct nice perfect congratulations all right i think at that point it just opens up questions to everybody else yeah it was a question everyone else so 8080 is the default port that tomcat runs on if you don't over write it just you're close kind of um so yeah at this point open up the questions that you have about anything that we covered today uh taking it beyond that in the networking side on the dev side anything and i'll see if there are any questions are there any questions on the stream oh sorry i'm totally connected i couldn't see it no um so so in fact way back when i was doing a tech before i got into different careers but does network engineering um kind of fall under the same thing as like network administration absolutely yeah they're very similar sometimes they'll just be called network administrators sometimes they'll be called network engineers or senior network engineers been around a little bit longer same thing with the senior network admin there's also network architects which are people who would be more in the design side and less on the administration side engineers sometimes bleed into both categories honestly it kind of just is up to the company that's making the job wreck what title they want to put on it so the original question i'm sorry was is like network engineer and network administrator related are they the same thing so and did you have a question back to me uh yeah i was going to ask the rsa algorithm that it uses to encrypt it is that similar to like shop256 they they're very similar although both end up getting used because sha is a hashing algorithm whereas rsa is like the actual it's the name of the whole suite that does the encryption so you have encryption algorithms inside of there too like aes or triple dez is the name of one there's a few different encryption algorithms there's a few different hashing algorithms and then all of that gets encompassed in rsa good question what was the question i'm sorry the question was um about was rsa and sha256 were they kind of related two of the same thing or yeah how they compared yeah are there any special considerations when building an application for use outside of the us cryptographic region yeah maybe not so for the actual application itself but any piece of hardware that is able to run encryption algorithms in general uh be that ssh or the https that we're looking at here that is dictated at a government level what is actually allowed and what the standards are that that has to be deployed to so in america i have to purchase gear from america that follows american encryption or cryptographic standards if i'm in some other country i need to purchase the gear from there and ensure it had followed whatever the standards are of that country i can't ship an extra server i have in america to another country that can run encryption algorithms um most likely if you even tried to do that it would just get intercepted and you just lose it and i didn't repeat that question again do i need to so the question was is are there any special considerations for when you're trying to deploy an encrypted or secure application um outside of your country sporters cool yeah uh you were using spring as like a fancy injection engine is there like a javascript for that um i'm not sure for uh for c-sharp i know there is um i don't know what it's called um the dot-net world um but in uh for javascript with node uh it's specifically an angular i know that you can use it's got dependency injection built in constructor based dependency injection i'm not sure if react has something like that but um to answer your question i believe there are packages you can add on that will do it but it's not as mature or commonly used to to to use dependency injection as far as a design pattern in a javascript application but good question so the question was uh is dependency injection used um is what is the equivalent of springs dependency injection engine used in javascript so yeah guys got any more questions anybody nothing online all right sweet well if there's nothing else i'd just really like to uh thank everyone again for attending um and look forward to seeing everyone uh in these future events so thanks again thank you inaudible
2021-11-17 12:05